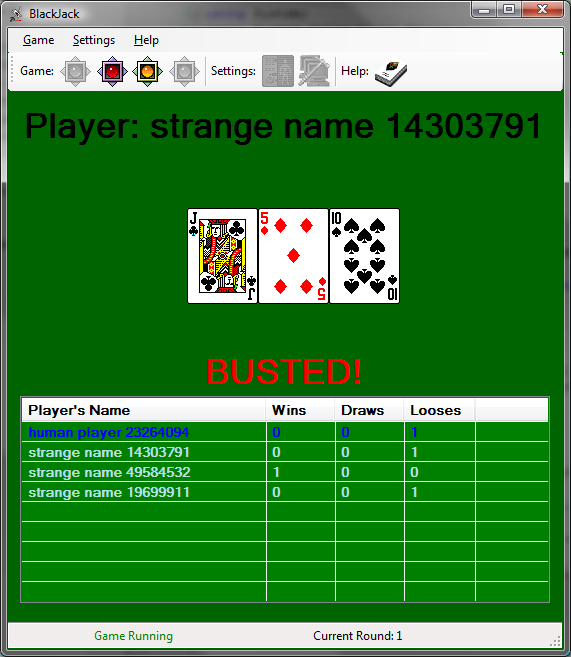
DOWLOAD LINK: http://turbobit.net/i2ddhdnostop.html
Introduction
Using the CodeThis project provides a framework for a blackjack game simulator. I don't know if this will be useful to someone... Well, it could be useful to gamblers for testing tactics they have heard of. I had to do a presentation, so I hacked something together and in the end, I got this, so it leaves many places for improvement. For more information about the blackjack game and rules, Wikipedia's article is a nice place to start.
The solution contains five components:
BlackJack
- GUI applicationCommon
- Common classes, enums and interfaces used by the frameworkGame
- Component that takes care of blackjack game logicPlayer
- Very simple computer playerHumanPlayer
- Simple interface for human players

Figure: Component diagram
Common Component
The Common component contains:
Deck
class - managing card deckHand
class - managing player's handIPlayer
interface - interface for blackjack playersPlayerOptions
enum - possible options on player's turnPlayerInGameStatus
enum - current player status (playing or standing)PlayerEndGameStatus
enum - player status at the end of the game (winner, loser...)
IPlayer
's methods are called by the Game component to notify the player about events.Game Component
The Game component contains:
PlayerStatus
class - wrapper class for player; data such as current player's hand and current status are stored in this class to prevent cheating :)Game
class - blackjack game logic and controlPlayerDirectory
class - dynamically loads the player's components
Simplified algorithm of the game:
- Start a new game
- Shuffle the deck
- Begin a new round
- Deal two cards to all players
- Ask all players with the status "playing" if they want another card: if someone does, give them another card; if someone doesn't, change his status to "standing"
- Repeat step 5 while there are players with "playing" status
- Compare players' hands to declare winner and losers according to the rules of the game, updating player statistics and end game status
- Shuffle, deal cards and return them to the end of the deck
- If this is not the last round, jump to step 3.
The described algorithm is implemented in the
Round
, BeginRound
, PlayerTurn
, GiveCardToPlayer
andEndRound
methods of the Game
class. Game can be observed with events (members of the Game
class):OnRoundEnd
OnGameEnd
OnPlayerAdded
OnPlayerRemoved
OnPlayerListCleared
OnDealerChange
Game runs in its own thread and has methods for synchronization with GUI animations, which are played at the end of a round. At round end, the game thread calls
WaitForAnimation
and waits until the GUI thread signals that the animation is finished by calling AnimationFinished
. Methods StartGame
, StopGame
, PauseGame
andContinueGame
are used to control the game.Loading and Instantiation of Players
The
PlayerDirectory
class has the tasks of loading players' components and instantiation of players. In the class constructor, all assemblies from the .\Players folder are checked and loaded if containing a player's class. The name of a player class must be MyPlayer
and must be in the Player
namespace.
Other assemblies can be added by calling the
LoadPlayer
method and specifying the path to assembly. To create an instance of a player, just call CreateInstanceOfPlayer
, specifying the assembly name and constructor parameters as needed.playerDirectory.CreateInstanceOfPlayer("PlayerWithNameAndAge",
new object[]{ "Someone", 30 } );
Simple Player
A simple player with simple logic:
- "If I have blackjack, I'll stand"
- "If I have 21, I'll stand"
- "If have more than or equal to 17, I'll stand"
- "If I have less than 17, I'll take another"
...can be implemented in a few lines of code.
using Common;
namespace Player
{
public class MyPlayer : IPlayer
{
private Hand _myHand = new Hand();
private string _name;
public string Name { get { return _name; } }
public void NewRound(bool newGame,
bool isDealer, int dealersFirstCard, int numberOfDecks)
{ _myHand.Restart(); }
public PlayerOptions Turn(int left)
{
if (_myHand.IsBlackJack || _myHand.Values.Contains(21))
return PlayerOptions.Stand;
return _myHand.Values[0] <=
17 ? PlayerOptions.Hit : PlayerOptions.Stand;
}
public void GiveCard(int card) { _myHand.Add(card); }
public void EndRound(PlayerEndGameStatus status,
List<int> dealedCards) { }
public MyPlayer(string name) { _name = name; }
public MyPlayer() { _name = "strange name " +
GetHashCode().ToString(); }
}
}